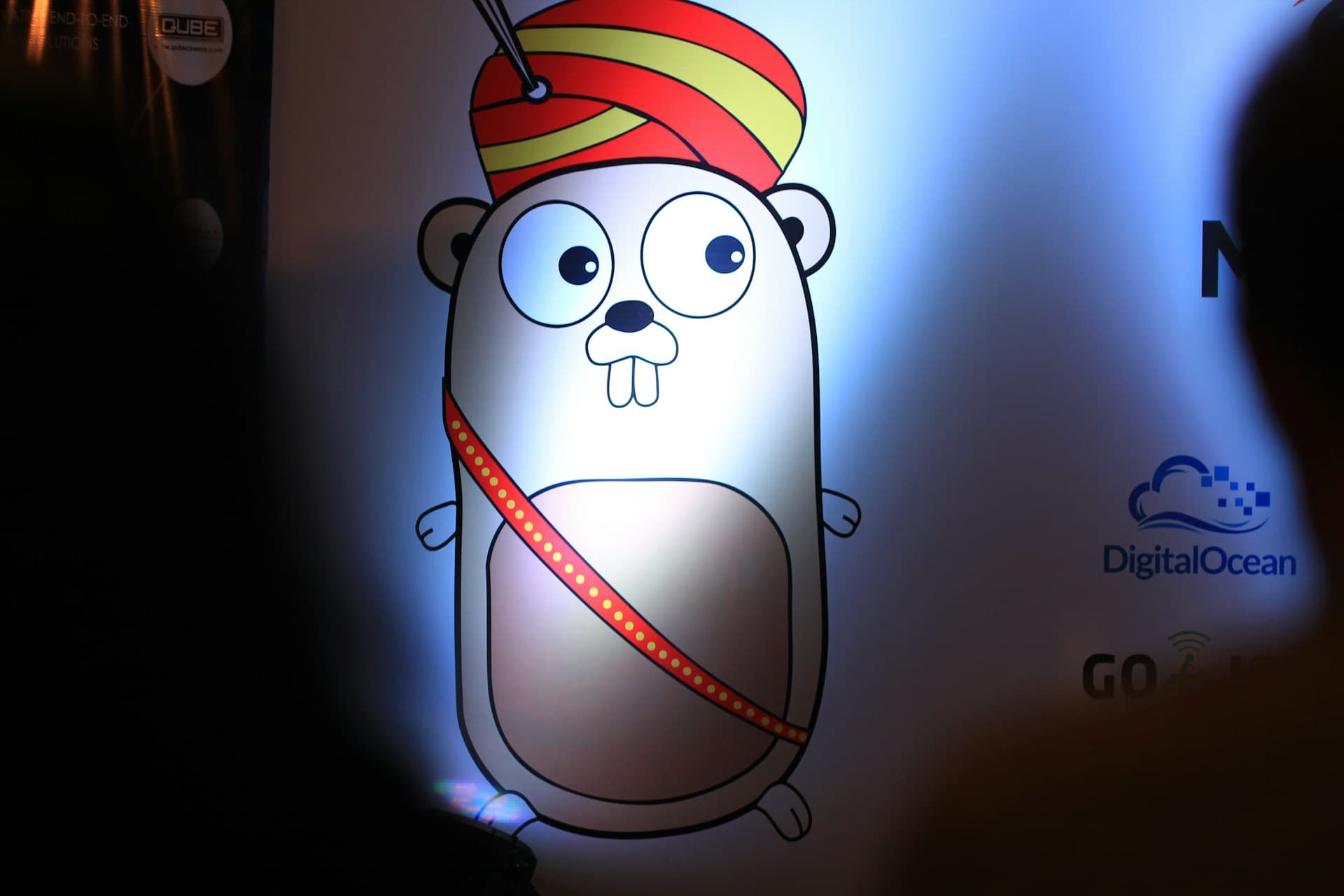
Advanced Golang Concurrency Patterns
Learn how to leverage Go's powerful concurrency features to build efficient applications.
Introduction to Golang Concurrency
Go (or Golang) was designed with concurrency as a first-class citizen. Unlike many other programming languages, Go provides built-in primitives that make concurrent programming more accessible and less error-prone.
The key to understanding Go's concurrency model lies in two core concepts:
- Goroutines - Lightweight threads managed by the Go runtime
- Channels - Communication mechanisms between goroutines
In this blog post, we'll explore advanced patterns that leverage these primitives to create robust concurrent applications.
Beyond Basic Goroutines
Let's start with a simple goroutine:
func main() {
go func() {
fmt.Println("Hello from a goroutine!")
}()
time.Sleep(1 * time.Second) // Wait for goroutine to finish
}
This works, but using time.Sleep
to wait for goroutines is not ideal. Let's look at better patterns.
The Worker Pool Pattern
One of the most useful concurrency patterns in Go is the worker pool. It allows you to process tasks concurrently while limiting the number of active goroutines.
func worker(id int, jobs <-chan int, results chan<- int) {
for j := range jobs {
fmt.Printf("Worker %d processing job %d\n", id, j)
time.Sleep(time.Second) // Simulate work
results <- j * 2
}
}
func main() {
const numJobs = 10
const numWorkers = 3
jobs := make(chan int, numJobs)
results := make(chan int, numJobs)
// Start workers
for w := 1; w <= numWorkers; w++ {
go worker(w, jobs, results)
}
// Send jobs
for j := 1; j <= numJobs; j++ {
jobs <- j
}
close(jobs)
// Collect results
for a := 1; a <= numJobs; a++ {
<-results
}
}
This pattern is incredibly powerful for tasks like processing items from a queue, handling HTTP requests, or any scenario where you want to limit concurrent execution.
Fan-Out, Fan-In Pattern
The fan-out, fan-in pattern is useful when you need to split work among multiple goroutines and then combine their results.
func generator(nums ...int) <-chan int {
out := make(chan int)
go func() {
for _, n := range nums {
out <- n
}
close(out)
}()
return out
}
func square(in <-chan int) <-chan int {
out := make(chan int)
go func() {
for n := range in {
out <- n * n
}
close(out)
}()
return out
}
func merge(cs ...<-chan int) <-chan int {
var wg sync.WaitGroup
out := make(chan int)
// Start an output goroutine for each input channel
output := func(c <-chan int) {
for n := range c {
out <- n
}
wg.Done()
}
wg.Add(len(cs))
for _, c := range cs {
go output(c)
}
// Start a goroutine to close out once all the output goroutines are done
go func() {
wg.Wait()
close(out)
}()
return out
}
func main() {
in := generator(1, 2, 3, 4, 5)
// Fan out - distribute the square work to multiple goroutines
c1 := square(in)
c2 := square(in)
c3 := square(in)
// Fan in - consume all results
for n := range merge(c1, c2, c3) {
fmt.Println(n)
}
}
Timeouts and Cancellation
In real-world applications, you often need to handle timeouts and cancellation. Go's context
package is perfect for this:
func doWork(ctx context.Context) <-chan int {
result := make(chan int)
go func() {
defer close(result)
// Simulate work that takes time
select {
case <-time.After(2 * time.Second):
result <- 42
case <-ctx.Done():
fmt.Println("Work cancelled:", ctx.Err())
return
}
}()
return result
}
func main() {
// Create a context with a timeout
ctx, cancel := context.WithTimeout(context.Background(), 1*time.Second)
defer cancel()
// Start the work
result := doWork(ctx)
// Wait for result or timeout
select {
case r := <-result:
fmt.Println("Result:", r)
case <-ctx.Done():
fmt.Println("Main:", ctx.Err())
}
}
Conclusion
Go's concurrency model makes it possible to write efficient, concurrent code without the complexity of traditional multi-threading. The patterns we've discussed here:
- Worker pools for controlled concurrency
- Fan-out, fan-in for parallelizing work
- Context for timeout and cancellation
These patterns form the foundation for building sophisticated concurrent applications in Go. By mastering these concepts, you'll be able to write Go code that effectively utilizes available resources while maintaining readability and safety.
Remember, the best concurrent code is often the simplest. Go's design encourages simple, understandable approaches to concurrency, so embrace these idioms rather than fighting against them.